![[정렬] 백준 10825번 : 국영수 - Java](https://img1.daumcdn.net/thumb/R750x0/?scode=mtistory2&fname=https%3A%2F%2Fblog.kakaocdn.net%2Fdn%2F2KKV5%2FbtsMTeJRUEA%2FU29Dyw0TRNHc1qKLL536O0%2Fimg.png)
[정렬] 백준 10825번 : 국영수 - Java백준/정렬2025. 3. 21. 15:52
Table of Contents
https://www.acmicpc.net/problem/10825
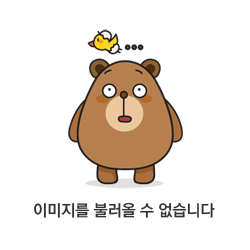
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
import java.util.ArrayList;
import java.util.Collections;
import java.util.StringTokenizer;
// 구현, 정렬
public class Main {
public static void main(String[] args) throws IOException {
BufferedReader br = new BufferedReader(new InputStreamReader(System.in));
int N = Integer.parseInt(br.readLine());
StringTokenizer st;
ArrayList<Student> myList = new ArrayList<>();
for (int i = 0; i < N; i++) {
st = new StringTokenizer(br.readLine());
String name = st.nextToken();
int kor = Integer.parseInt(st.nextToken());
int eng = Integer.parseInt(st.nextToken());
int math = Integer.parseInt(st.nextToken());
myList.add(new Student(name, kor, eng, math));
}
Collections.sort(myList);
for (int i = 0; i < myList.size(); i++) {
System.out.println(myList.get(i).name);
}
}
}
class Student implements Comparable<Student> {
String name;
int kor;
int eng;
int math;
public Student(String name, int kor, int eng, int math) {
this.name = name;
this.kor = kor;
this.eng = eng;
this.math = math;
}
// 국어 감소, 영어 증가, 수학 감소, 이름 사전순
// 정렬 기준을 나타내는 함수
// 0보다 작으면 현재 객체가 o보다 작음 (내림차순)
// 0이면 두 객체가 같음
// 0보다 크면 현재 객체가 o보다 큼 (오름차순)
@Override
public int compareTo(Student o) {
if (this.kor != o.kor) {
return o.kor - this.kor; // 내림차순 (-)
} else if (this.eng != o.eng) {
return this.eng - o.eng; // 오름차순 (+)
} else if (this.math != o.math) {
return o.math - this.math; // 내림차순 (-)
}
return this.name.compareTo(o.name); // 국영수 점수가 같다면 이름을 사전순으로 비교
}
}
@킴준현 :: 차근차근 꾸준히
포스팅이 좋았다면 "좋아요❤️" 또는 "구독👍🏻" 해주세요!